2.4. If Statements#
The if
statement lets us execute a section of code if a specified condition
is True.
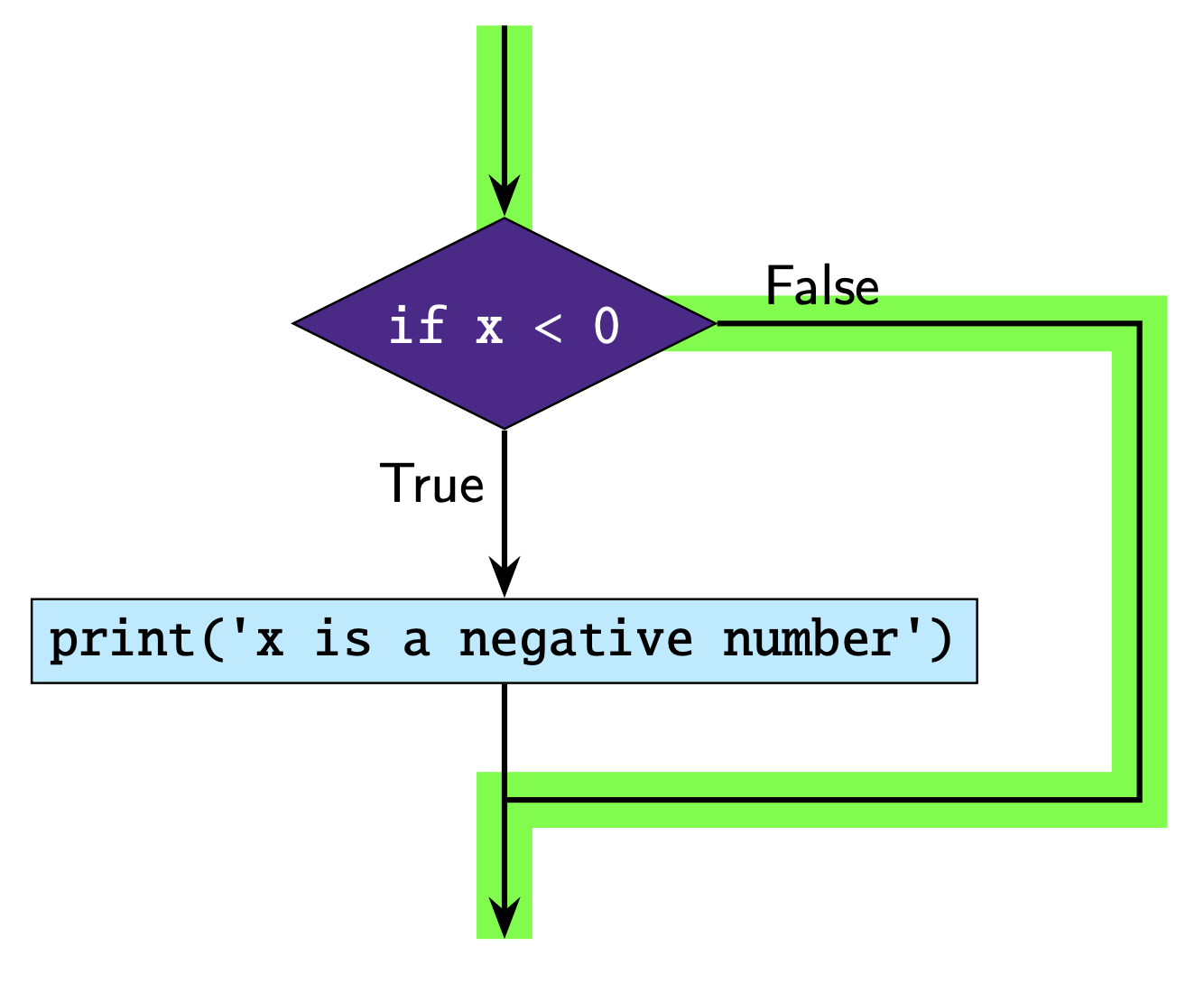
The structure of an if statement is:
if condition:
# code you execute if condition is true
Take note of the following:
if
is a keywordThe condition must evaluate to either
True
orFalse
The code inside the if statement only executes if the condition is
True
:
is placed at the end of the conditionThe code inside the if statement must be indented. The indentation defines the code block. This allows you to put multiple lines inside the if statement.
The indentation can be done using tab or spaces, as long as you’re consistent!
Here is an example of a simple if statement.
x = -2
if x < 0:
print('x is a negative number')
x is a negative number
In this example the condition is True
so the print statement runs.
This is how we can represent this code diagrammatically. The green line indicates the ‘path’ the code takes.
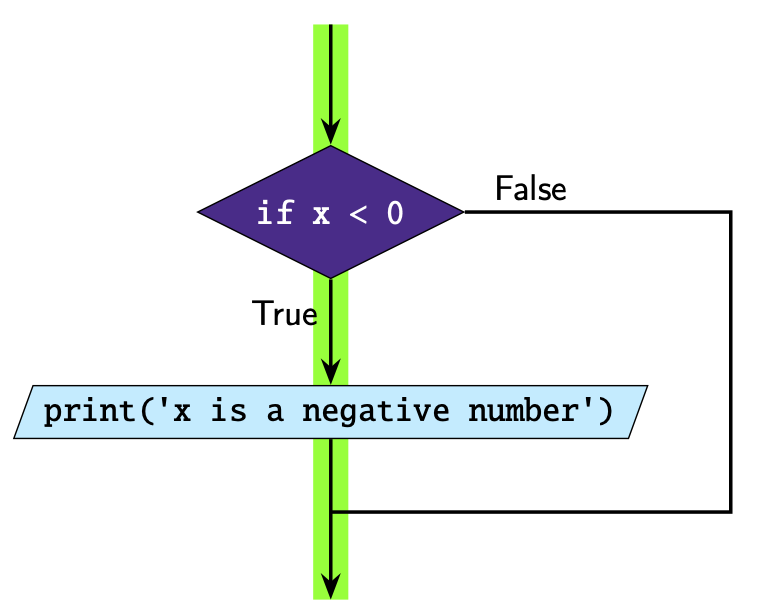
Let’s look at another example.
x = 1
if x < 0:
print('x is a negative number')
In this example the condition is False
so the print statement does not run.
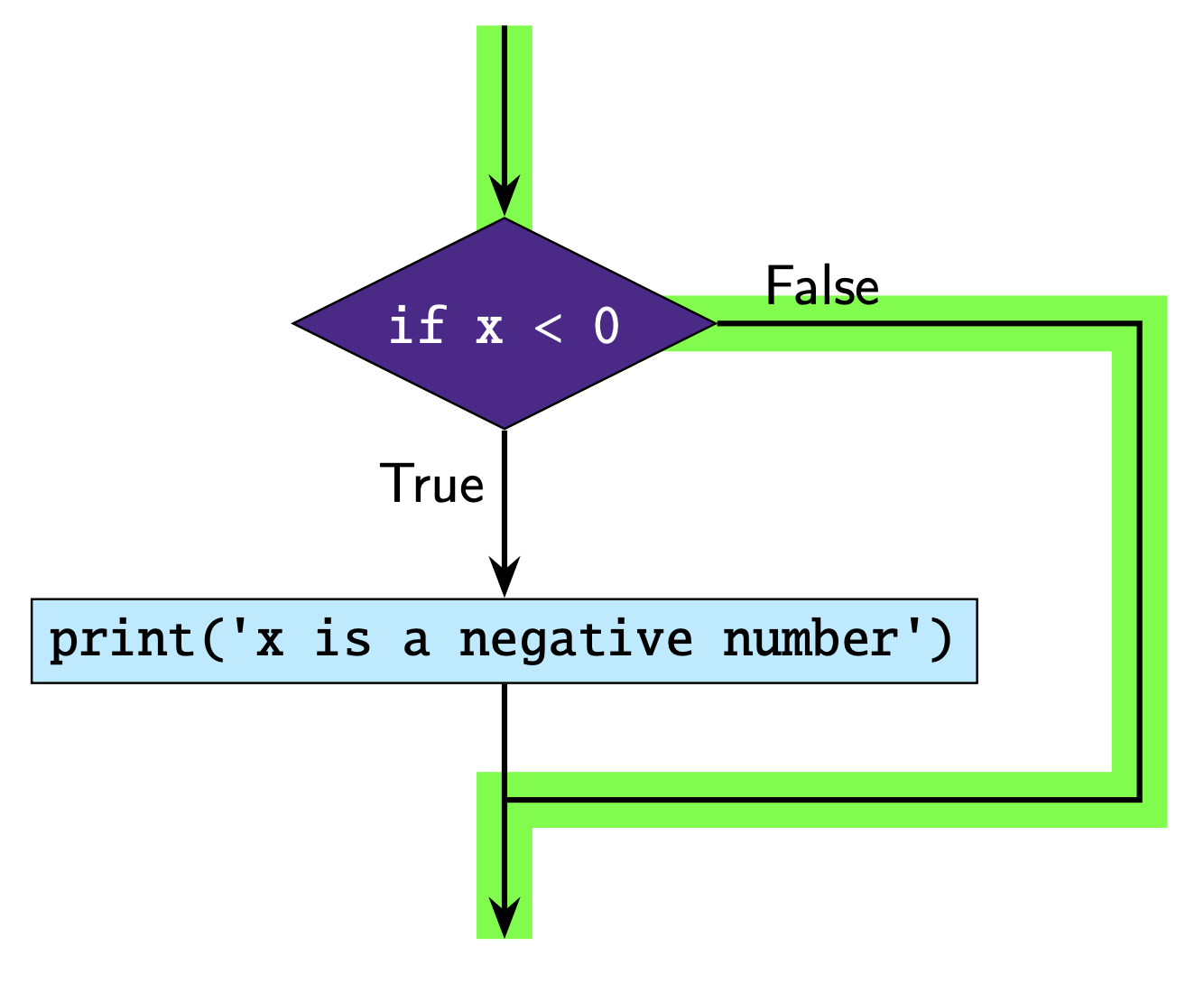
Question 1
What do you think the output of the following code will be?
weather = 'rainy'
if weather == 'rainy':
print('Take an umbrella!')
if weather == 'sunny':
print('Take a hat!')
Question 2
What do you think the output of the following code will be?
status = 'still cooking'
if status == 'finished':
print('Cake is done.')
print('Take it out of the oven!')
print('Yum cake!')
Solution
Solution is locked
Code challenge: Big Number
Write a program that reads in a number from the user. If that number is greater than 100 your program should output:
That's a big number!
Example 1
Enter a number: 107
That's a big number!
Example 2
Enter a number: 2
Solution
Solution is locked
Code challenge: 3 is Lucky!
Write a program that asks the user for a number. If that number is 3, the program should say 3 is lucky! If the user enters any other number, the program should say nothing.
Example 1
Enter a number: 3
3 is lucky!
Example 2
Enter a number: 5
Solution
Solution is locked
Code challenge: Favourite Colour
Write a program that asks for the user’s favourite colour. If their favourite colour is red, the program should output:
That's my favourite colour!
Regardless of what colour the user says, the program should also say:
What a nice colour
Here are some examples of how your code should run.
Example 1
What is your favourite colour? red
That's my favourite colour!
What a nice colour
Example 2
What is your favourite colour? blue
What a nice colour
Hint
Don’t forget to take note of the spelling!
Solution
Solution is locked
Code challenge: Temperature
Write a program that reads in today’s temperature (in degrees Celsius) from the user.
If the temperature is less than 15 degrees the program should output:
Take a jumper!
If the temperature is greater than 25 degrees the program should output:
Take a fan.
You can assume all temperatures are given to the nearest degree.
Example 1
What's the temperature like today? 5
Take a jumper!
Example 2
What's the temperature like today? 30
Take a fan.
Example 3
What's the temperature like today? 20
Solution
Solution is locked