2.6. If-Elif-Else
Statements#
To add more conditions to an if
-else
statement, we can use elif
.
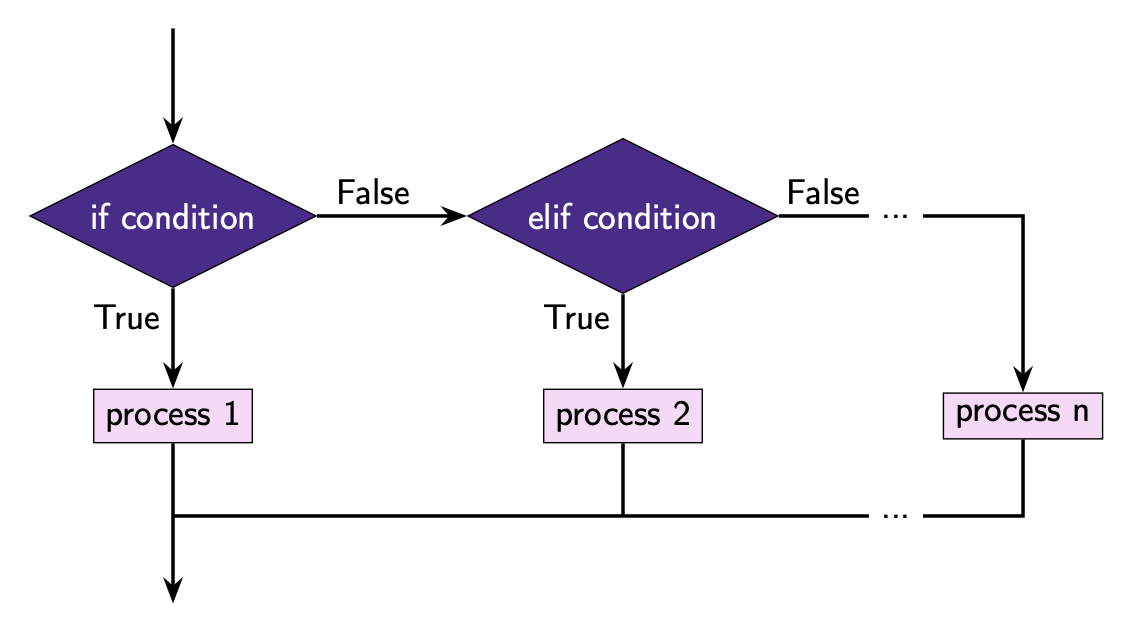
The elif
statement must be used between the if
and the else
statements. You can also have as many elif
statements as you want!
Here is how we use the elif
statement:
if condition_1:
# code to execute if condition 1 is true
elif condition_2:
# code to execute if condition 2 is true
elif condition_3:
# code to execute if condition 3 is true
...
else:
# code to execute if none of the conditions are true
Take note of the following:
elif
is a keywordEach condition must evaluate to either
True
orFalse
:
is placed at the end of each conditionThe first condition that evaluates to
True
controls which section of code executesThe code inside the
elif
statement must be indented. Note that only the code blocks are indented. The lines beginning withif
,elif
orelse
are not indented.
Here is an example of an if
-elif
-else
statement.
x = 1
if x < 0:
print("x is a negative number")
elif x > 0:
print("x is a positive number")
else:
print("x is 0!")
In this example the second condition is True
so the second print
statement runs.
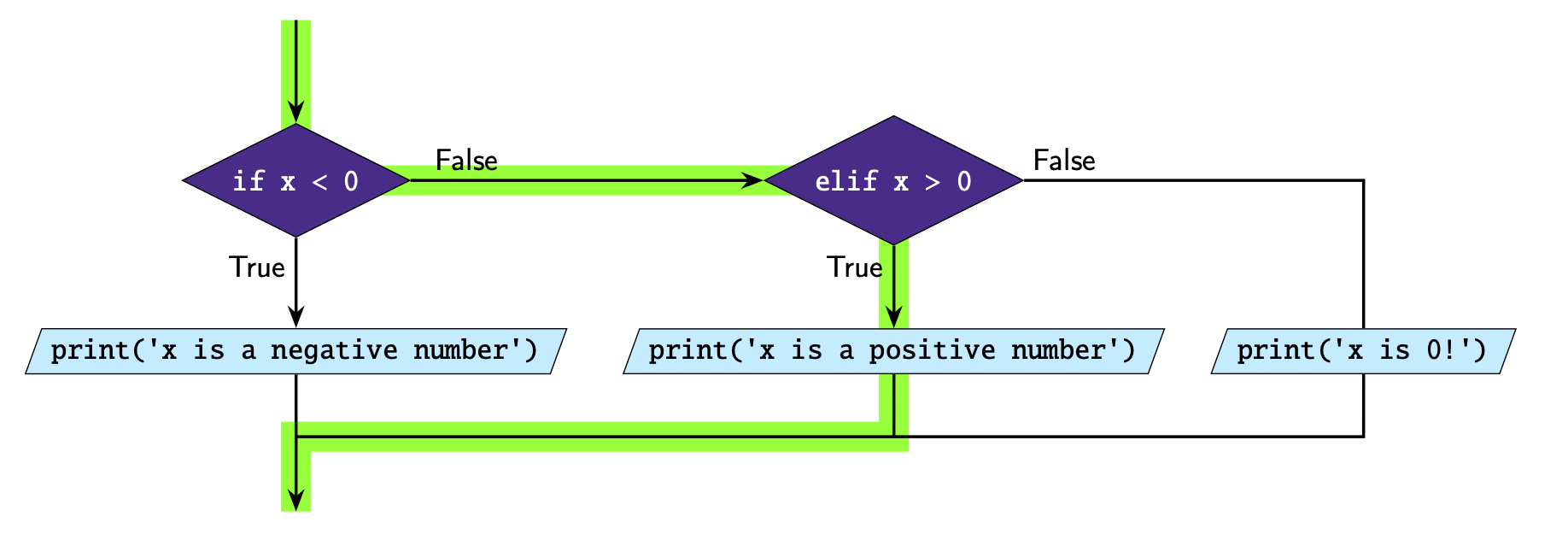
Question 1
What do you think the output of the following code will be?
light = 'green'
if light == 'green':
print('GO!')
elif light == 'yellow':
print('Slow down!')
elif light == 'red':
print('Stop!')
else:
print("That can't be right. Traffic lights can't be {}.".format(light))
Question 2
Consider the two programs below. They are similar but not quite the same. If you set the variable light
to 'yellow'
, both programs will print Slow down!.
Program 1
light = 'yellow'
if light == 'green':
print('GO!')
if light == 'yellow':
print('Slow down!')
if light == 'red':
print('Stop!')
Program 2
light = 'yellow'
if light == 'green':
print('GO!')
elif light == 'yellow':
print('Slow down!')
elif light == 'red':
print('Stop!')
else:
print("That can't be right. Traffic lights can't be {}.".format(light))
Suppose we changed the value stored in the variable light
. For which of the following values of light
will program 1 and program 2 result in different outputs? Select all that apply.
light = 'green'
light = 'yellow'
light = 'red'
light = 'blue'
light = 'purple'
Solution
Solution is locked