2.5. If-Else Statements#
If you want to execute code if a condition is False
, we can use the
else
statement.
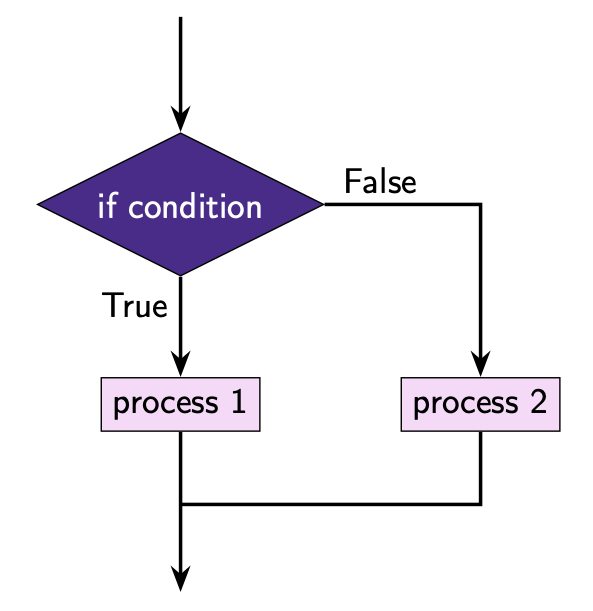
The else
statement is always used after an if
statement. The
structure of an if
-else
statement is:
if condition:
# code you execute if condition is true
else:
# code you execute if condition is false
Take note of the following:
else
is a keywordThe code inside the
else
statement only executes if the condition isFalse
. Theelse
statement doesn’t have a condition after it. (Remember: Else is lonely. Else is by itself!):
is placed after theelse
Similar to the
if
statement, the code inside theelse
statement must be indented.
Here is an example of a simple if
-else
statement.
x = 1
if x < 0:
print("x is a negative number")
else:
print("x is a positive number")
In this example the condition is False so the second print statement runs.
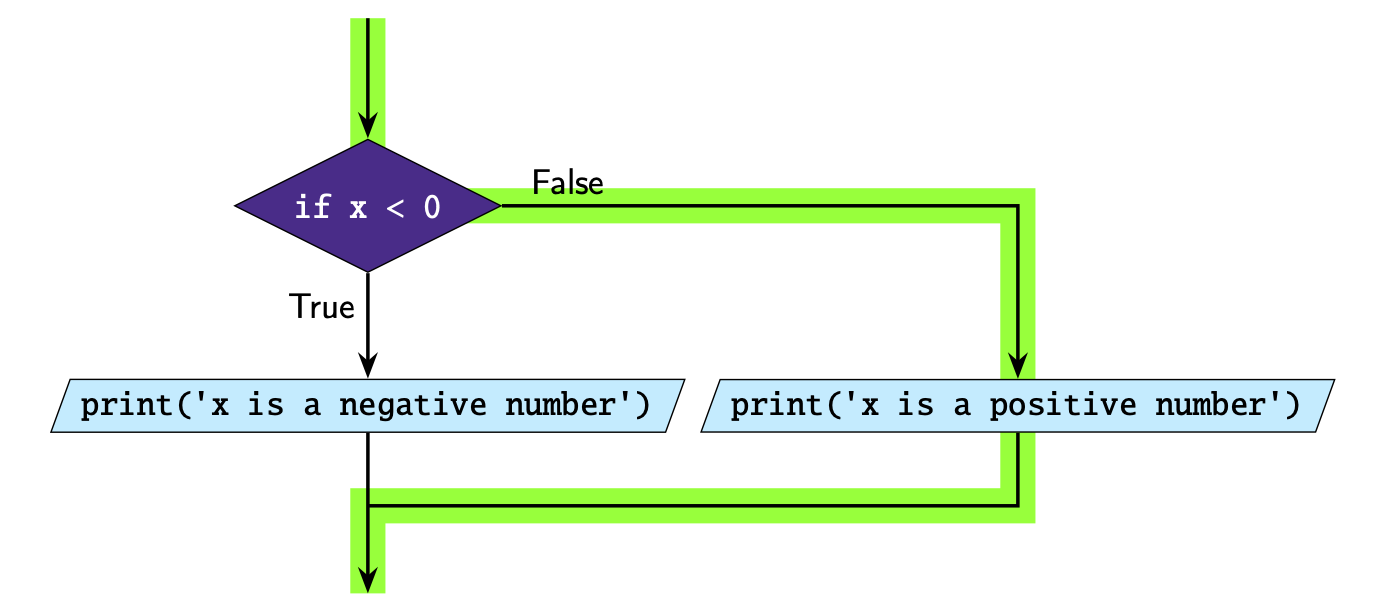
Question 1
What do you think the output of the following code will be?
current_speed = 45
speed_limit = 40
if current_speed > speed_limit:
print('You are speeding! {}km/h is above the speed limit of {}km/h. Slow down!'.format(current_speed, speed_limit))
else:
print('You are travelling within the speed limit.')
Question 2
What do you think the output of the following code will be?
time = 6
print("I'm hungry.")
if time == 6:
print("It's dinner time!")
else:
print('Dinner will be soon...')
print('I hope we get pizza for dinner!')
Solution
Solution is locked
Code challenge: Elevator
Code challenge: Odd Even
Write a program that asks the user for an integer and then displays whether the number is odd or even.
Example 1
Enter a number: 1
This number is odd
Example 2
Enter a number: 2
This number is even
Hint
Use the modulus operator %
to returns the remainder.
When even numbers are divided by 2 the remainder is 0
When odd numbers are divided by 2 the remainder is 0
Solution
Solution is locked