2.8. Pseudocode#
Sometimes it can be useful to write algorithms in code form without the syntax of a specific language. We can do this using pseudocode. This is a way of writing code in a format that is easy for a human to read. The exact symbols and keywords may vary depending on the convention you use.
The way in which we represent if
-elif
-else
statements as follows.
This is an example with 3 conditions.
IF condition A THEN
process 1
ELSEIF condition B THEN
process 2
ELSEIF condition C THEN
process 3
ELSE
process 4
ENDIF
Here’s an example!
Python code
x = 1
if x < 0:
print('x is a negative number')
elif x > 0:
print('x is a positive number')
else:
print('x is 0!')
Pseudocode
IF x < 0 THEN
Display 'x is a negative number'
ELSEIF x > 0 THEN
Display 'x is a positive number'
ELSE
Display 'x is 0!'
ENDIF
Question 1
Which of the following provides the pseudocode that corresponds to the following Python program?
weather = 'rainy'
if weather == 'rainy':
print('Take an umbrella!')
IF weather == rainy: Display 'Take an umbrella'
IF 'Take an umbrella' weather == 'rainy' ENDIF
ELSEIF weather == 'rainy' Display 'Take an umbrella' ENDELSE
IF weather == 'rainy' THEN Display 'Take an umbrella' END IF
Solution
IF weather == rainy:
Display 'Take an umbrella'
Invalid. Uses :
instead of THEN
and missing ENDIF
at the end.
IF 'Take an umbrella'
weather == 'rainy'
ENDIF
Invalid and Incorrect. Here the condition and the process have been swapped. The IF
is also missing the THEN
.
ELSEIF weather == 'rainy'
Display 'Take an umbrella'
ENDELSE
Invalid. The first condition should be an IF
not an ELSEIF
. The closing tag should be ENDIF
instead of ENDELSE
IF weather == 'rainy' THEN
Display 'Take an umbrella'
END IF
Correct.
Question 2
Which of the following provides the pseudocode that corresponds to the algorithm illustrated below?
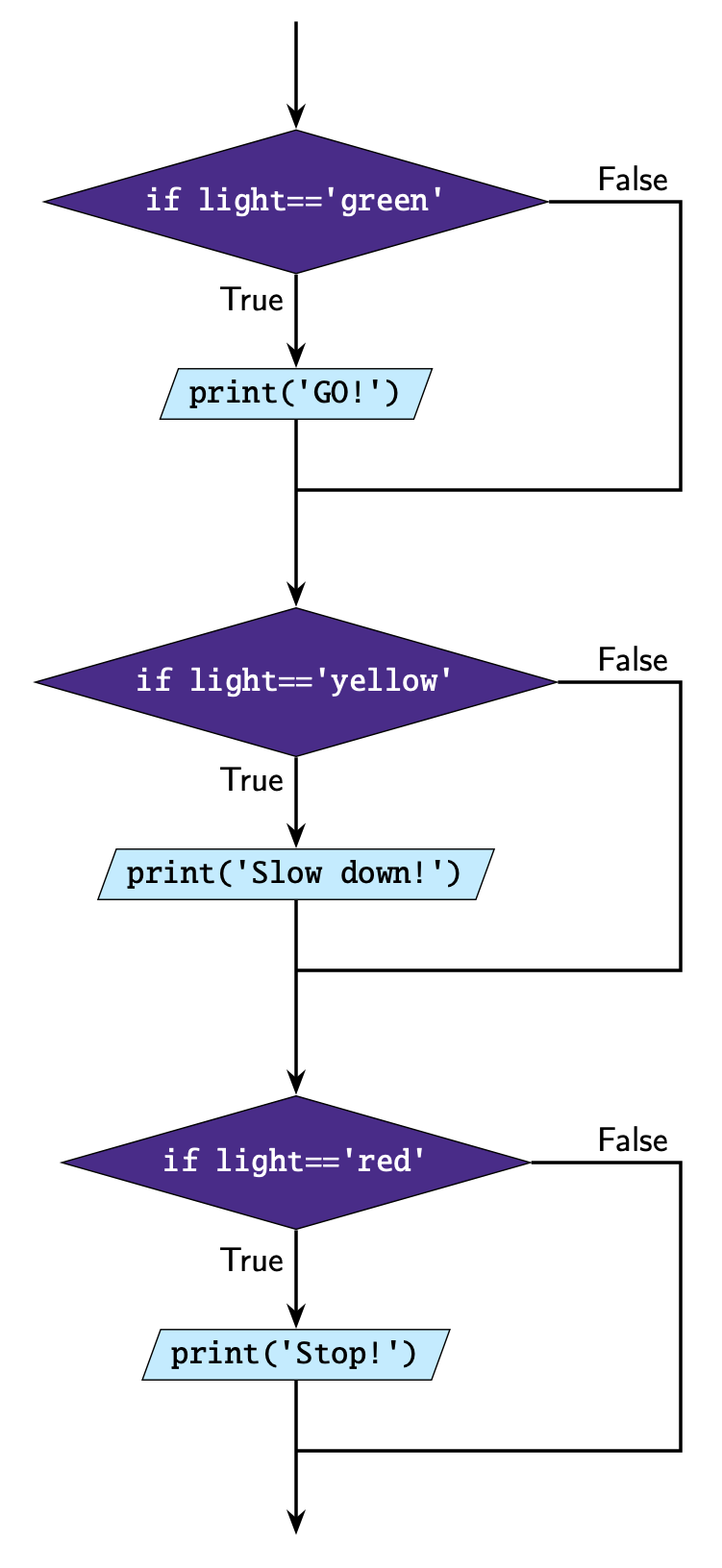
IF light == 'green' THEN Display 'GO!' ELSEIF light == 'yellow' THEN Display 'Slow down!' ELSEIF light == 'red' THEN Display 'Stop!' ENDIF
IF light == 'green' THEN Display 'GO!' ELSEIF light == 'yellow' THEN Display 'Slow down!' ELSE Display 'Stop!' ENDIF
IF light == 'green' THEN Display 'GO!' ELSE IF light == 'yellow' THEN Display 'Slow down!' ELSE IF light == 'red' THEN Display 'Stop!' ELSE
IF light == 'green' THEN Display 'GO!' ENDIF IF light == 'yellow' THEN Display 'Slow down!' ENDIF IF light == 'red' THEN Display 'Stop!' ENDIF
Solution
Solution is locked
Code challenge: Starting Player
The following algorithm is used to pick a starting player. Both players roll a die and whoever rolls the largest number gets to start. If it’s a tie, then the younger player starts. If it’s a tie again then player 2 starts.
IF roll1 > roll2 THEN
Display 'Player 1 starts'
ELIF roll2 > roll1 THEN
Display 'Player 2 starts'
ELSE
IF age1 < age2 THEN
Display 'Player 1 starts'
ELSE
Display 'Player 2 starts'
Write the Python code that corresponds to the given pseudocode.
Solution
Solution is locked