4.4. Random Floats and Using Probabilities#
Sometimes we want an event to occur with a specific probability. This can be
achieved using random.random()
. This function produces a random float
between 0 (inclusive) and 1 (not inclusive), i.e. we will get a value \(r\)
where \(0 \leq r < 1\).
import random
print(random.random())
We can then check whether the random value is less than or equal to \(p\), the probability with which we want the event to occur.
For example, we can write a program to generate weather conditions, which is common in a lot of games. Our simple program will say that it’s sunny 80% of the time and say that it’s rainy 20% of the time.
import random
r = random.random()
if r < 0.8:
print("sunny")
else:
print("rainy")
Question 1
Which of the following values could you obtain after running the following program? Select all that apply.
import random
r = random.random()
print(r*10)
0
1
10
0.5
0.368479
7.8
Solution
r = random.random()
will produce a random float between 0 (included) and 1 (not included). Since these values get multiplied by 10, we end up with values between 0 (included) and 10 (not included).
Question 2
Write a program that will generate floats between -1 (included) and 1 (not included), i.e. will print \(r\) where \(-1 \leq r < 1\).
Solution
Solution is locked
Question 3
Which of the following best describes the purpose of the code shown below.
import random
r = random.random()
if r < 0.6:
print('Heads')
else:
print('Tails')
The program simulates a fair coin which will present Heads 50% of the time and Tails 50% of the time.
The program simulates an unfair coin which will present Heads 60% of the time and Tails 40% of the time.
The program simulates an unfair coin which will present Heads 40% of the time and Tails 60% of the time.
Question 4
Write a program that simulates a loaded dice with the following probabilities.
1, 2, 3, 4, 5 each appear 15% of the time
6 appears 25% of the time
Solution
Solution is locked
Code challenge: Fitness Wheel
Write a program that allows the user to spin the fitness wheel!
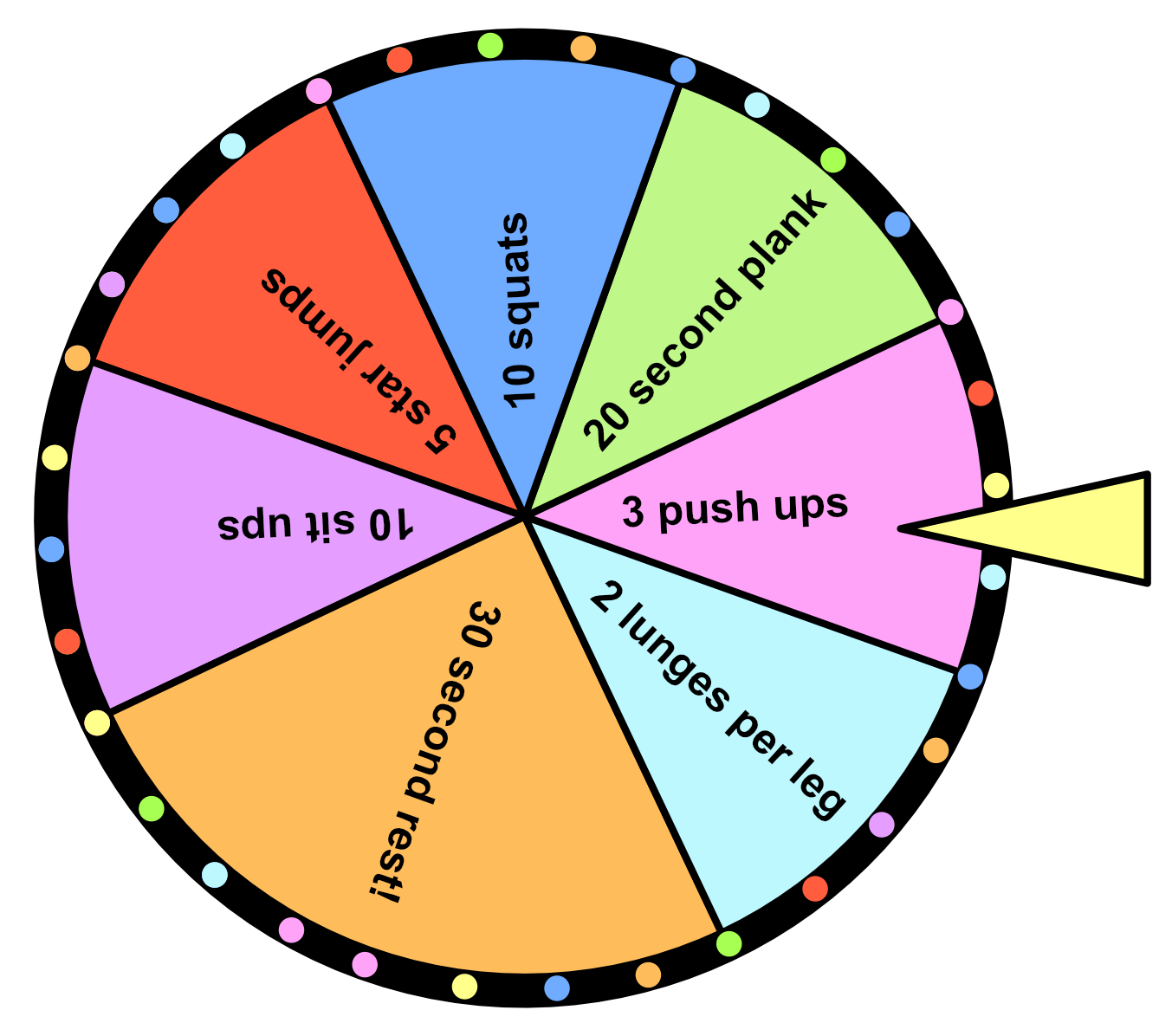
The program should display
10 sit ups 12.5% of the time
5 star jumps 12.5% of the time
10 squats 12.5% of the time
20 second plank 12.5% of the time
3 push ups 12.5% of the time
2 lunges per leg 12.5% of the time
30 second rest! 25% of the time
Solution
Solution is locked