3.13. Range#
When using for
loops, you have seen that we typically loop through a list.
We can automatically create lists of sequential values using the range()
function. The range()
function creates an iterable with a specific set of
numbers.
The structure of the range()
function is:
range(end)
range(start, end)
range(start, end, step_size)
Note
The start value is included while the end value is excluded and the start, end, and step_size must be integers.
To see exactly what the range()
function produces, it’s easiest to convert
the result to a list using the list()
function. Let’s see some examples.
Example range(end):
end value is specified. By default, range()
will start at 0, and count up in steps of 1 to the end value, but does not
include the end value.
print(list(range(3)))
[0, 1, 2]
Example range(start, end):
start and end value is specified.
range()
will start at the specified start value, and count up in steps of 1
to the end value, but does not include the end value.
print(list(range(2, 5)))
[2, 3, 4]
Example range(start, end, step):
start and end value is specified.
range()
will start at the specified start value, and count up by the
specified step to the end value, but does not include the end value.
print(list(range(6, 15, 3)))
[6, 9, 12]
You can also specify a negative step, which means range will count down. The
same rules apply as previously in that range()
will start at the specified
start value, and count down by the specified step to the end value, but does
not include the end value.
print(list(range(10, 2, -2)))
[10, 8, 6, 4]
Here is an example of what range()
might look like in a for
loop.
for i in range(5):
print(i)
0
1
2
3
4
Note that this is effectively the same as
for i in [0, 1, 2, 3, 4]:
print(i)
0
1
2
3
4
or
i = 0
while i < 5:
print(i)
i = i + 1
0
1
2
3
4
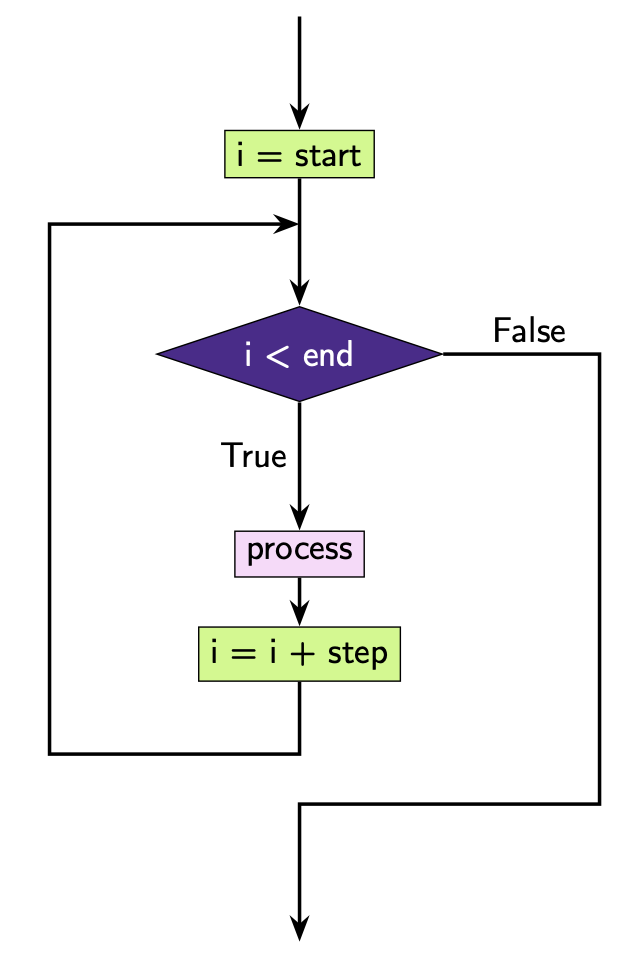
Since we know what values the iteration variable will take in a for
loop
using range (we know the start, the end and step), we can represent
it using a flow chart similar to how we draw flowcharts for while loops.
Question 1
What do you think the output of the following code will be?
print(list(range(5, 11)))
[5, 6, 7, 8, 9, 10, 11]
[5, 6, 7, 8, 9, 10]
[6, 7, 8, 9, 10, 11]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
Solution
Recall that range(start, end, step)
means range()
will start at the specified start value, and count up by the specified step to the end value, but does not include the end value. This means we’ll start at 5 and count up to but not include 11.
Question 2
Rewrite the following code using range()
.
total = 0
for i in [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]:
total = total + i
print(total)
Solution
Solution is locked
Question 3
Which of the following are equivalent to range(0, 5, 1)
? Select all that apply.
[0, 1, 2, 3, 4, 5]
range(0, 5)
range(5, 1)
list(range(5)
Solution
Solution is locked
Question 4
What do you think the output of the following code will be?
for i in range(3):
print('Hello!')
1 Hello! 2 Hello! 3 Hello!
0 Hello! 1 Hello! 2 Hello!
Hello! Hello! Hello!
Hello!
Question 5
Given the following list
musicals = ['Wicked', 'Les Miserables', 'Hamilton', 'Cats', 'Phantom of the Opera']
Write a for
loop that will result in the following output
1. Wicked
2. Les Miserables
3. Hamilton
4. Cats
5. Phantom of the Opera
Solution
Solution is locked
Code challenge: Count Up
Write a program that reads in an integer, and counts from 0 up to that integer. Your program should use a for loop.
Example 1
Enter a number: 3
0
1
2
3
Example 2
Enter a number: 5
0
1
2
3
4
5
Solution
Solution is locked