4.17. Pseudocode and Flowcharts#
4.17.1. Pseudocode#
When representing a function in pseudocode we use the keywords BEGIN
and
END
to indicate the start and end of the function.
BEGIN function_name
process
END function_name
If the function returns a value we use the keyword RETURN
followed by the
values that are returned
RETURN values
Here’s an example.
Python code
def add(x, y):
return x + y
Pseudocode
BEGIN add (x, y)
RETURN x + y
END add (x, y)
Once you have the pseudocode for a function, you can use this function in other
scripts. For your main script you can simply use BEGIN
and END
.
Python code
def add(x, y):
return x + y
for i in range(3):
doubled = add(i, i)
print(doubled)
Pseudocode
BEGIN
FOR i = 0 TO 2 STEP 1
doubled = add(i. i)
Display doubled
END
BEGIN add (x, y)
RETURN x + y
END add (x, y)
4.17.2. Flowcharts#
We now introduce two new shapes. The terminator and the subprocess.

Terminator - oval: Used to indicate the start and end of a program.
Subprocess - bordered rectangle: Used to refer to another program
Here’s an example.
Python code
def add(x, y):
return x + y
for i in range(3):
doubled = add(i, i)
print(doubled)
Flow chart
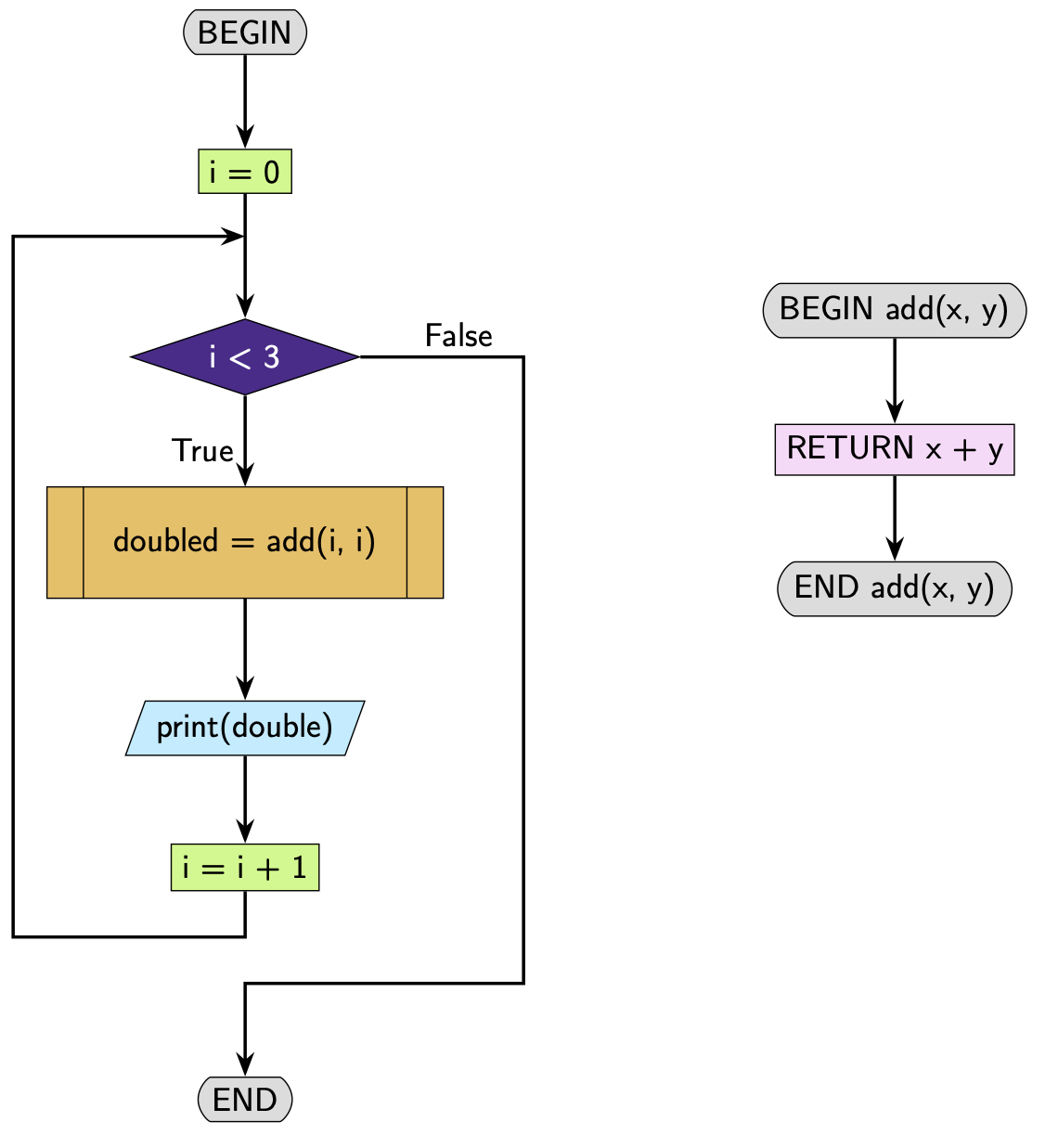
Note that there are two flow charts here. The first shows the main script
and the second shows the function add()
. The main script refers to the
add()
function in the subprocess node.
We have now seen all the flowchart symbols we will need.

Note that the colours are arbitrary and what’s important are the shapes.

Question 1
Which of the following programs corresponds to the pseudocode shown below?
BEGIN
my_numbers = [5, -2, 0, 4, 0, -3, -3]
description = []
FOR i = 0 TO Length(my_numbers) - 1 STEP 1
d = sign(my_numbers[i])
Append d to description
NEXT i
Display description
END
BEGIN sign(x)
IF x < 0 THEN
RETURN 'negative'
ELIF x > 0 THEN
RETURN 'positive'
ELSE
RETURN '0'
END sign(x)
def my_numbers(5, -2, 0, 4, 0, -3, -3): description = [] for i in range(len(my_numbers)): description.append(my_numbers[i]) return description sign(x) if x < 0: return 'negative' elif x > 0: return 'positive' else: return '0'
def my_numbers(5, -2, 0, 4, 0, -3, -3): description = [] for i in range(len(my_numbers)): description.append(my_numbers[i]) return description def sign(x): if x < 0: return 'negative' elif x > 0: return 'positive' else: return '0'
def sign(x): if x < 0: print('negative') elif x > 0: print('positive') else: print('0') my_numbers = [5, -2, 0, 4, 0, -3, -3] description = [] for i in range(len(my_numbers)): description.append(sign(my_numbers[i])) print(description)
def sign(x): if x < 0: return 'negative' elif x > 0: return 'positive' else: return '0' my_numbers = [5, -2, 0, 4, 0, -3, -3] description = [] for i in range(len(my_numbers)): description.append(sign(my_numbers[i])) print(description)
Solution
Incorrect. In the pseudocode there is a function called
sign(x)
. You can tell from the pseudocode because there is aBEGIN sign(x)
and anEND sign(x)
. There is nosign(x)
function in the python code.Incorrect. In the pseudocode there is no function
my_numbers(5, -2, 0, 4, 0, -3, -3)
. This is a variable created in the main script. You can identify the main script because it has aBEGIN
andEND
without a function name. this python code has a function called`` my_numbers``.Incorrect.
sign()
should containreturn
statements, notprint
statements. The pseudocode hasreturn
statements.Correct.
Question 2
Which of the following flowcharts corresponds to the pseudocode shown below?
BEGIN
my_numbers = [5, -2, 0, 4, 0, -3, -3]
description = []
FOR i = 0 TO Length(my_numbers) - 1 STEP 1
d = sign(my_numbers[i])
Append d to description
NEXT i
Display description
END
BEGIN sign(x)
IF x < 0 THEN
RETURN 'negative'
ELIF x > 0 THEN
RETURN 'positive'
ELSE
RETURN '0'
END sign(x)
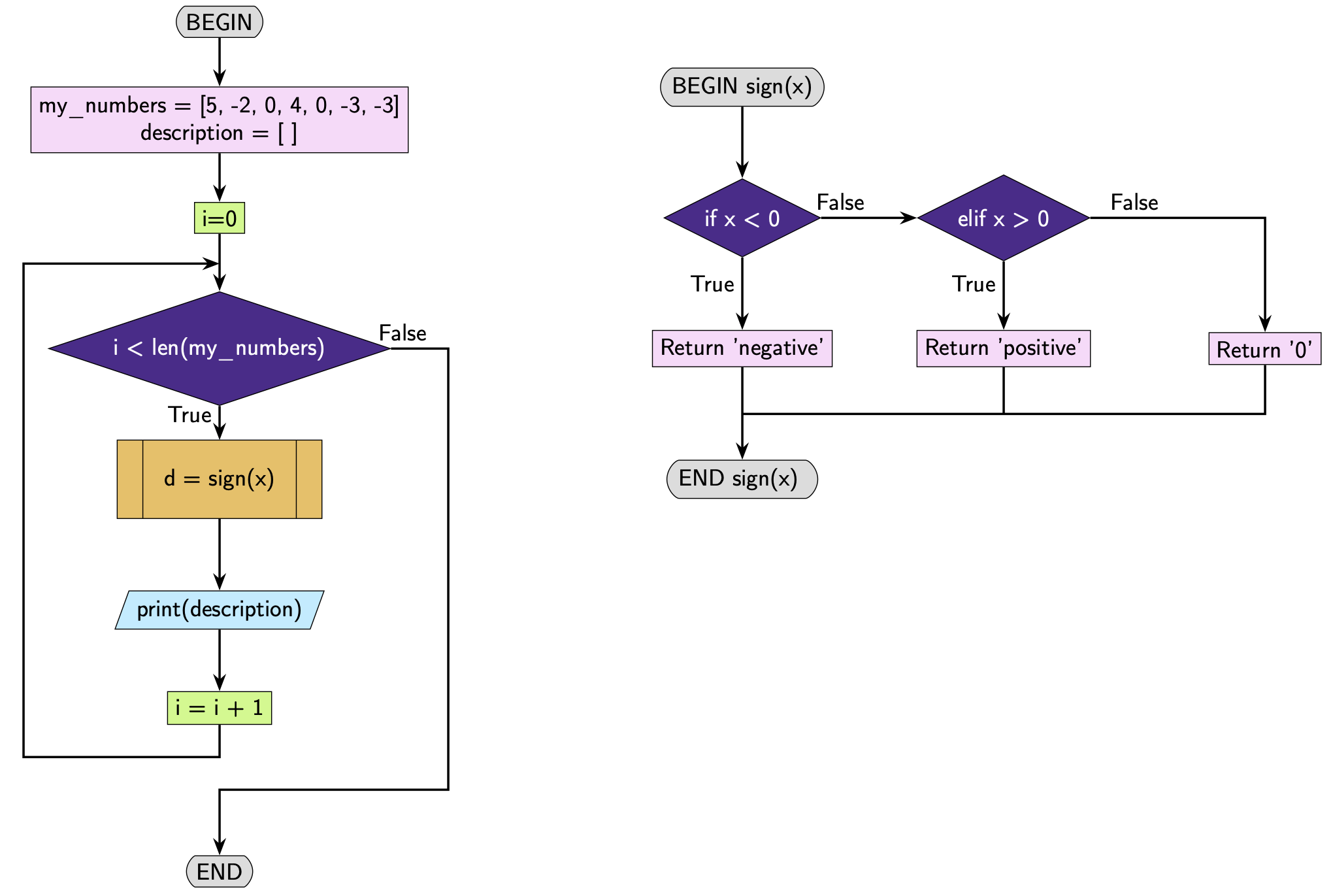
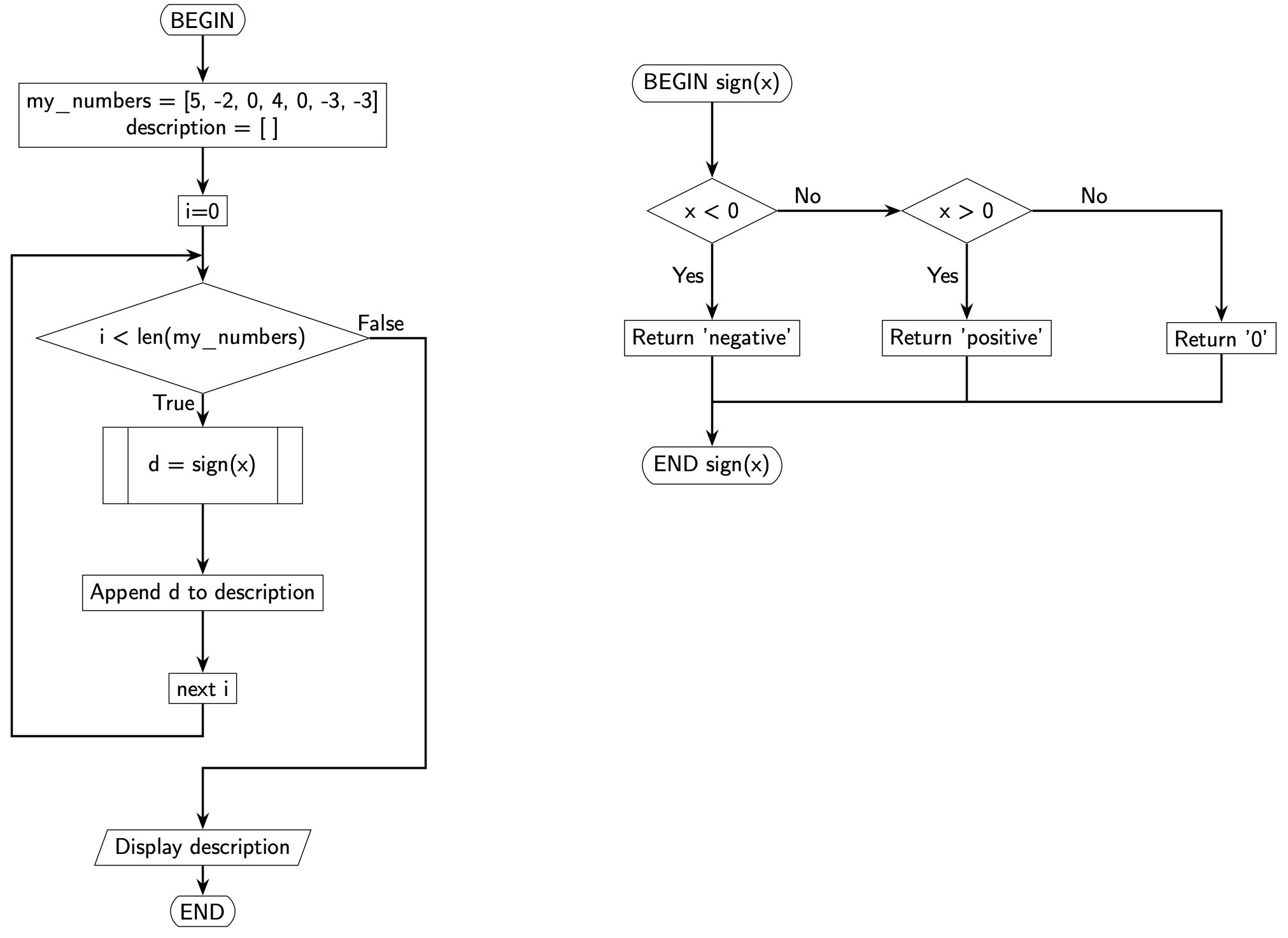
Solution
Solution is locked
Question 3
Construct the pseudocode that corresponds to the following Python function.
def zero_nonzero(number_list):
zero = 0
nonzero = 0
for i in range(len(number_list)):
if number_list[i] == 0:
zero = zero + 1
else:
nonzero = nonzero + 1
return zero, nonzero
Solution
Solution is locked